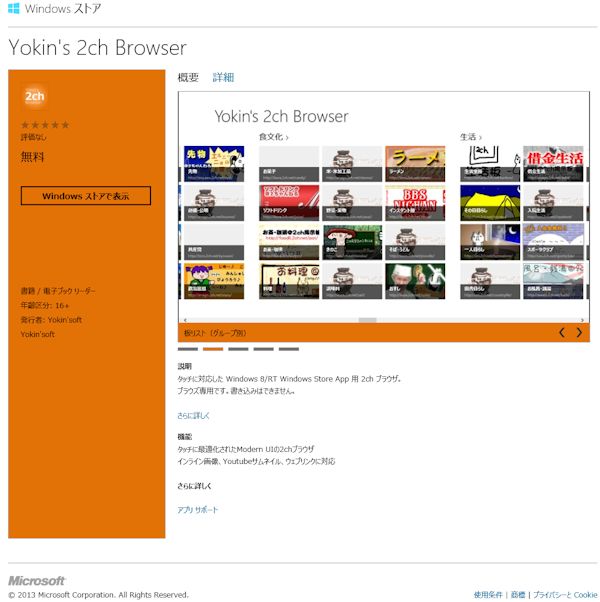
2015年3月から2ch専用ブラウザに関して2ch運営元の方針変更により当アプリはWindowsストアから撤去する予定です。ご利用ありがとうございました。
Windows 8/RT のいわゆるストアアプリ (Windows Store App)のプログラミングから登録手続きまでの流れの学習を兼ねて作成しました。2chリーダーです。アプリストアで検索したところでは、いまだ2chリーダーは登録されていないようでしたので、気合を入れて作ってみました。Androidでは 2chMate にいつもお世話になっているので、仕様は2chMateを強く意識した作りにしました。
http://apps.microsoft.com/windows/ja-JP/app/yokins-2ch-browser/203ac772-a869-4c3d-ac09-0e5d9b4499a1
主な実装済みの機能は
近く実装したい機能は
- スレッドのソート
- 記事のソート機能(タグによる参照順など)
- IDによるフィルター
- 各種設定画面の追加
いずれ必要な機能は
各所でお世話になっていることもあり、アプリ自体はフリーですが、Microsoft Advertising の広告を使用しています。Microsoftアプリストアを通じてダウンロード数や、ユーザーの反響などが得られるようなので、そちらを通じても、応援いただけたら機能追加の励みになります。
11. January 2013
Jake
ソフトウェア開発
.NETの標準以外に Newtonsoft のJSON.NETを使用します。http://james.newtonking.com/pages/json-net.aspx
使い方は
var container = new Google.GoogleCloudMessaging.Container {
RegistrationIds = new String [] { "APA*******PQZQ" },
Data = new { message = "Hello World!" }
};
var response = Google.GoogleCloudMessaging.Broadcast( "AIz************", container );
のようになります。
------------------------------
using System;
using System.Net;
using Newtonsoft.Json;
namespace Google
{
public class GoogleCloudMessaging
{
public class Container
{
[JsonProperty("registration_ids")]
public String[] RegistrationIds { get; set; }
[JsonProperty("collapse_key", NullValueHandling=NullValueHandling.Ignore )]
public String CollapseKey { get; set; }
[JsonProperty("data", NullValueHandling = NullValueHandling.Ignore)]
public object Data { get; set; }
[JsonProperty("delay_while_idle", NullValueHandling = NullValueHandling.Ignore)]
public Boolean? DelayWhileIdle { get; set; }
[JsonProperty("time_to_live", NullValueHandling = NullValueHandling.Ignore)]
public int? TimeToLive { get; set; }
[JsonProperty("restricted_package_name", NullValueHandling = NullValueHandling.Ignore)]
public String RestrictedPackageName { get; set; }
[JsonProperty("dry_run", NullValueHandling = NullValueHandling.Ignore)]
public Boolean? DryRun { get; set; }
}
public class Response
{
[JsonProperty("multicast_id")]
public String MulticastId { get; set; }
[JsonProperty("success")]
public int Success { get; set; }
[JsonProperty("failure")]
public int Failure { get; set; }
[JsonProperty("canonical_ids")]
public int CanonicalIds { get; set; }
[JsonProperty("results")]
public Result[] Results { get; set; }
}
public class Result
{
[JsonProperty("message_id")]
public String MessageId { get; set; }
[JsonProperty("registration_id")]
public String RegistrationId { get; set; }
[JsonProperty("error")]
public String Error { get; set; }
}
static public Response Broadcast(String API_KEY, Container container)
{
var request = WebRequest.Create("https://android.googleapis.com/gcm/send");
var json = Newtonsoft.Json.JsonConvert.SerializeObject(container);
using( WebClient web = new WebClient()){
web.Headers.Add("Authorization", "key=" + API_KEY );
web.Headers.Add("Content-Type", "application/json");
byte[] result = web.UploadData("https://android.googleapis.com/gcm/send", "POST", System.Text.Encoding.UTF8.GetBytes(json) );
String resultString = System.Text.Encoding.UTF8.GetString(result);
var response = Newtonsoft.Json.JsonConvert.DeserializeObject<Response>( resultString );
return response;
}
}
}
}